Axis Aligned Bounding Boxes and Intersections
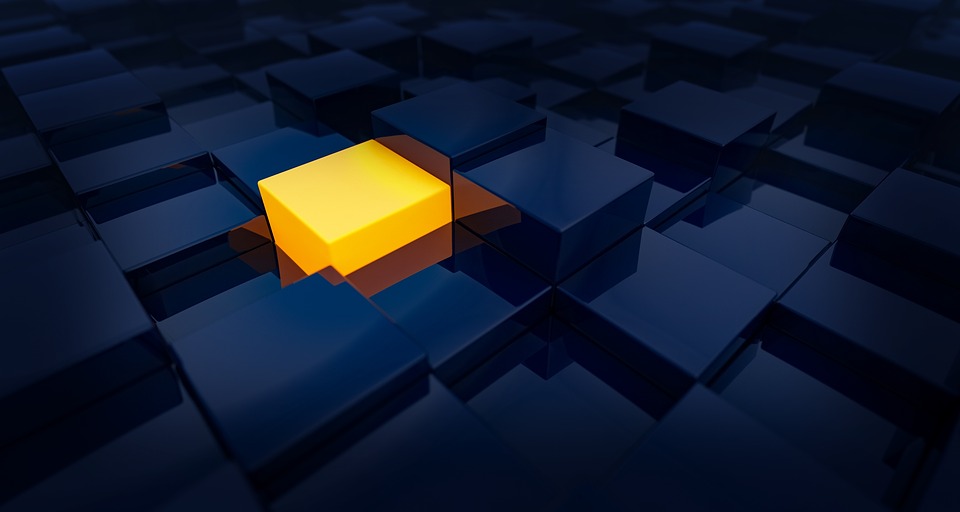
Let’s do a quick overview of AABBs and intersections.
Axis Aligned Bounding Box is a set of three segments (or 6 numbers). AABBs can be used for fast overlapping tests.
In one dimension it is a Segment
or AxisAlignedBounds1D
:
struct Segment {
public float min;
public float max;
}
Let’s calculate an intersection for two segments.
Segment Intersect(Segment a, Segment b) =>
new Segment {
Min = Math.Max(a.Min, b.Min),
Max = Math.Min(a.Max, b.Max)
};
In two dimensions it is a Rectangle
or AxisAlignedBounds2D
:
struct Rectangle {
Segment boundsX;
Segment boundsY;
}
Let’s calculate an intersection for two rectangles.
Rectangle Intersect(Rectangle a, Rectangle b) =>
new Rectangle {
X = Segment.Intersect(a.X, b.X),
Y = Segment.Intersect(a.Y, b.Y)
};
In three dimensions it is AABB
(Axis Aligned Bounding Box) or AxisAlignedBounds3D
:
struct AABB {
public Segment boundsX;
public Segment boundsY;
public Segment boundsZ;
}
Let’s calculate an intersection for two AABBs.
AABB Intersect(AABB a, AABB b) =>
new AABB {
X = Segment.Intersect(a.X, b.X),
Y = Segment.Intersect(a.Y, b.Y),
Z = Segment.Intersect(a.Z, b.Z),
};
Let’s do it slightly differently for fun.
Testing intersection via bounds in 1D:
struct Bounds{
public float Min;
public float Max;
public Bounds Intersection(Bounds other) =>
new Bounds {
Min = Math.Max(this.Min, other.Min),
Max = Math.Min(this.Max, other.Max)
};
public bool Intersects(Bounds other) {
var i = this.Intersection(other);
return i.Min < i.Max;
}
}
Testing intersections via bounds in 2D:
struct Bounds2D {
public Bounds BoundsX;
public Bounds BoundsY;
public Bounds2D Intersection(Bounds2D other) =>
new Bounds2D {
BoundsX = this.XBounds.Intersection(other.BoundsX),
BoundsY = this.YBounds.Intersection(other.BoundsY);
};
public bool IsValid => BoundsX.IsValid && BoundsY.IsValid;
public bool Intersects(Bounds2D other) =>
this.Intersection(other).IsValid;
}
Testing intersections via bounds in 3D:
struct Bounds3D {
public Bounds BoundsX;
public Bounds BoundsY;
public Bounds BoundsZ;
public Bounds3D Intersection(Bounds3D other) =>
new Bounds3D {
BoundsX = this.BoundsX.Intersection(other.BoundsX),
BoundsY = this.BoundsY.Intersection(other.BoundsY);
BoundsZ = this.BoundsZ.Intersection(other.BoundsZ);
};
public bool IsValid => BoundsX.IsValid && BoundsY.IsValid && BoundsZ.IsValid;
public bool Intersects(Bounds3D other) =>
this.Intersection(other).IsValid;
}