Data Oriented Design
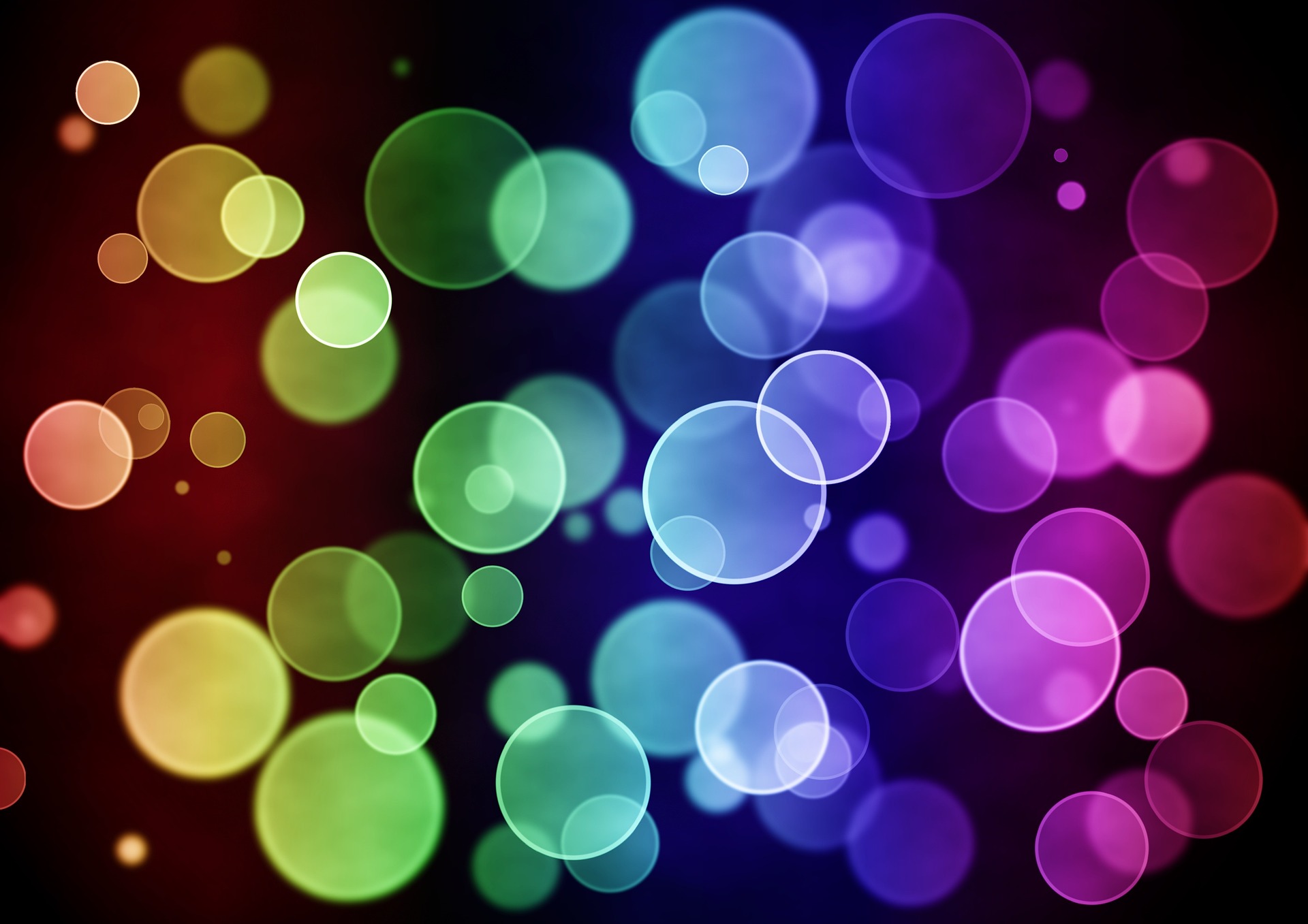
A few notes on Data Oriented Design.
- Study the data access patterns to determine where performance bottlenecks are.
- Group things together that are used together.
- Avoid creating dependencies between properties.
- Split infrequently accessed data as it's own thing.
Traditional Object Oriented Approach:
// Single object, everything grouped together.
class Circle {
Point Position;
Color Color;
float Radius;
void Draw() {}
}
And you would store the Circle instances in a collection.
Data Oriented Design:
// Data that is used frequently together is grouped together.
class Body {
Point Position;
float Radius;
}
// Contains all the implied, non-explicit, circles.
class Circles {
List<Body> Bodies;
List<Color> Color;
void Draw() {}
}
In this design there's no single Circle, just a bunch of implied circles. Operations can be run on the the circles at the same time. There's a separation of functionality. When you want to process an OO Circle you get all it's properties all at once even if you don't need them. In DO design the data that is used together is grouped together.
Results:
- Less cache misses.
- Improved performance in many cases.