Getting GodotXUnit up and Running with Rider
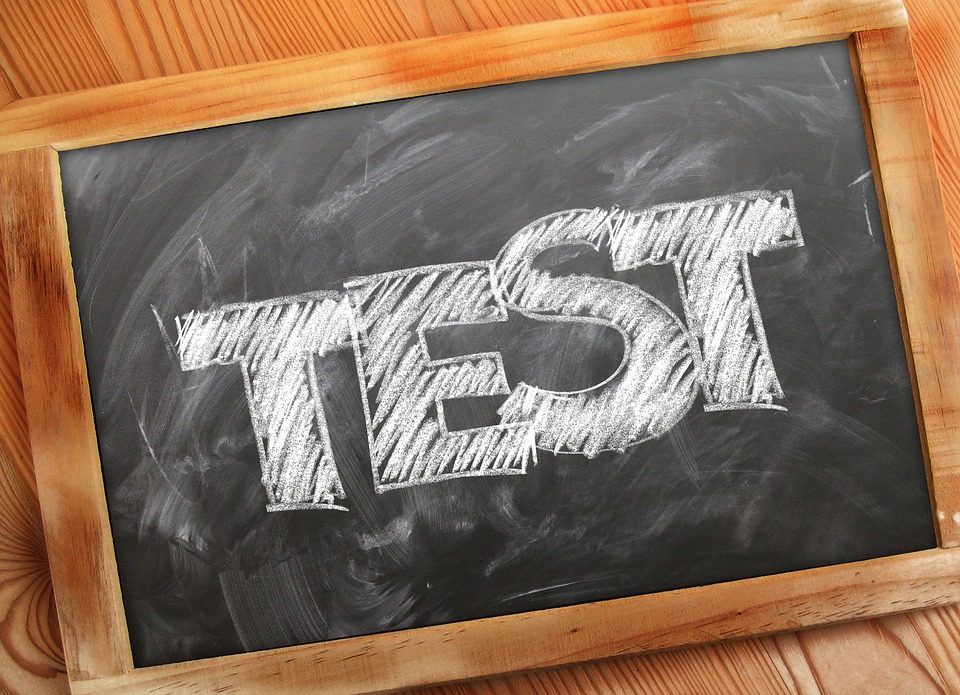
Installation from Github
These instructions will explain how to get GodotXUnit integrated to your project and working with Rider.
- Clone the
Master
branch of the GodotXUnit project in some temporary folder:git clone https://github.com/fledware/GodotXUnit.git
- From the freshly cloned project, copy the contents of the
addons
folder into your project’saddons
folder - or if that doesn’t exist yet then simply copy the wholeaddons
folder itself to your Godot project’s root. After doing this you can delete the rest of the files from the cloned project, you don’t need them. - Delete any
.csproj
files you can find underaddons/GodotXUnit
and its sub-folders. At the time of writing this there should be only one.csproj
located ataddons/GodotXUnit/GodotXUnitApi/GodotXUnitApi.csproj
. Delete it. With this installation method the GodotXUnit will become a part of your own project, so there’s no need to have any other.csproj
files lying around. - In the file
addons/GodotXUnit/GodotXUnitApi/GodotFactAttribute.cs
find a line that says:
[XunitTestCaseDiscoverer("GodotXUnitApi.Internal.GodotFactDiscoverer", "GodotXUnitApi")]
And replace the GodotXUnitApi
with your project’s assembly name, eg.
[XunitTestCaseDiscoverer("GodotXUnitApi.Internal.GodotFactDiscoverer", "MyAwesomeGodotProject")]
You have to do this because GodotXUnit will scan your project for test cases and it needs to know the assembly to scan. If you don’t do this GodotXUnit will not find your test cases.
- Add these dependencies to your project’s
.csproj
<ItemGroup>
<PackageReference Include="Newtonsoft.Json" Version="12.0.3" />
<PackageReference Include="xunit" Version="2.4.1" />
<PackageReference Include="xunit.runner.utility" Version="2.4.1" />
</ItemGroup>
Now you should be good to go.
Running the tests
Annotate your tests with [GodotFact]
and they should work directly from Rider.
If you want to write anything that uses the Godot Engine, make sure you make a base class for these tests and annotate it with a Collection attribute, as shown in the example below.
// super important - this prevents the tests from running in parallel.
// if they would run in parallel, they would interfere with each other.
[Collection("Integration Tests")] public abstract class BaseTest : IAsyncLifetime
And when waiting for things:
// Then within 5 seconds the player should be dead because
// the monster will attack the player.
await GetTree().WithinSeconds(5, () => {
// This assertion will be repeatedly run every frame
// until it either succeeds or the 5 seconds have elapsed.
Assert.True(arena.Player.IsDead);
});
Generally you can follow any instructions you can find for XUnit. The only real difference aside from the cases above is that you use [GodotFact]
instead of [Fact]
.
More information
Check out the GodotXUnit Github page: https://github.com/fledware/GodotXUnit