Inheritance with Constructors
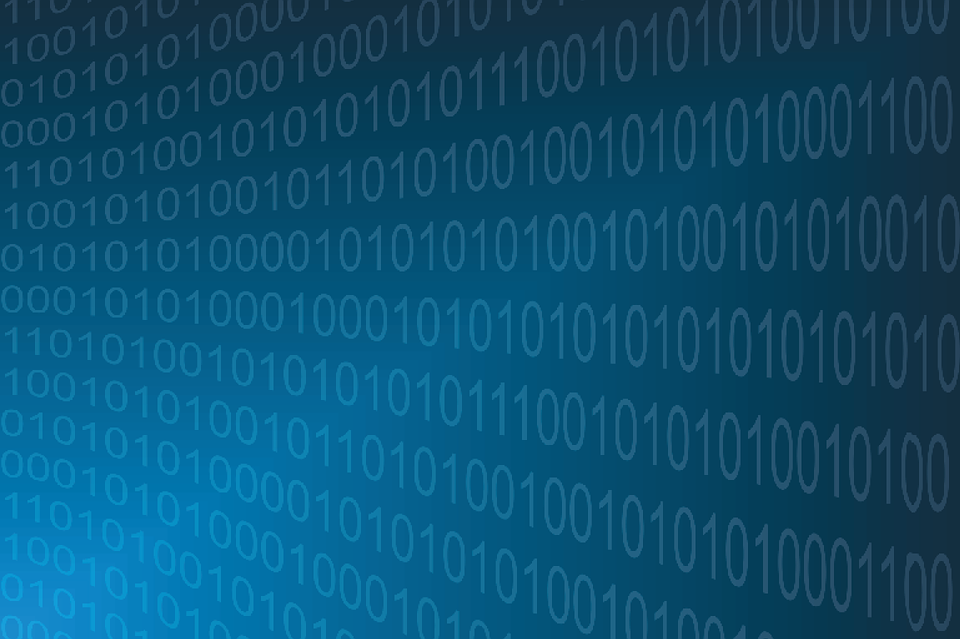
Here we call the constructor of the base class A and pass the id
as a parameter to initialize _id
in the constructor of A class.
By doing this way we can avoid unnecessary code duplication.
internal class A {
string _id;
internal A(string id) => _id = id;
}
internal class B : A {
string _name;
internal B(string name) : base(id) => _name = name;
}
Here we call another constructor inside the same class and create a new Author
object from the data provided to the first constructor. This is useful in cases where you already have an object constructed which you want to reuse.
internal class C {
Author _author;
string _message;
internal C(Author author, string message) {
_author = author;
_message = message;
}
internal C(string id, string name, string message) : this(new Author(id, name), message) {}
}
Passing in default values when default values cannot be used.
This example will not compile, but this is what we’d like to do.
public class D {
public D(string[] myArray = new string[] {"Entry1", "Entry2"}) {}
}
We can make it work by passing a faked default value.
public class E {
public E(string[] myArray) {}
public E() : this(new string[] {"Entry1", "Entry2"}) {}
}