Localizing Godot's default Splash Screen
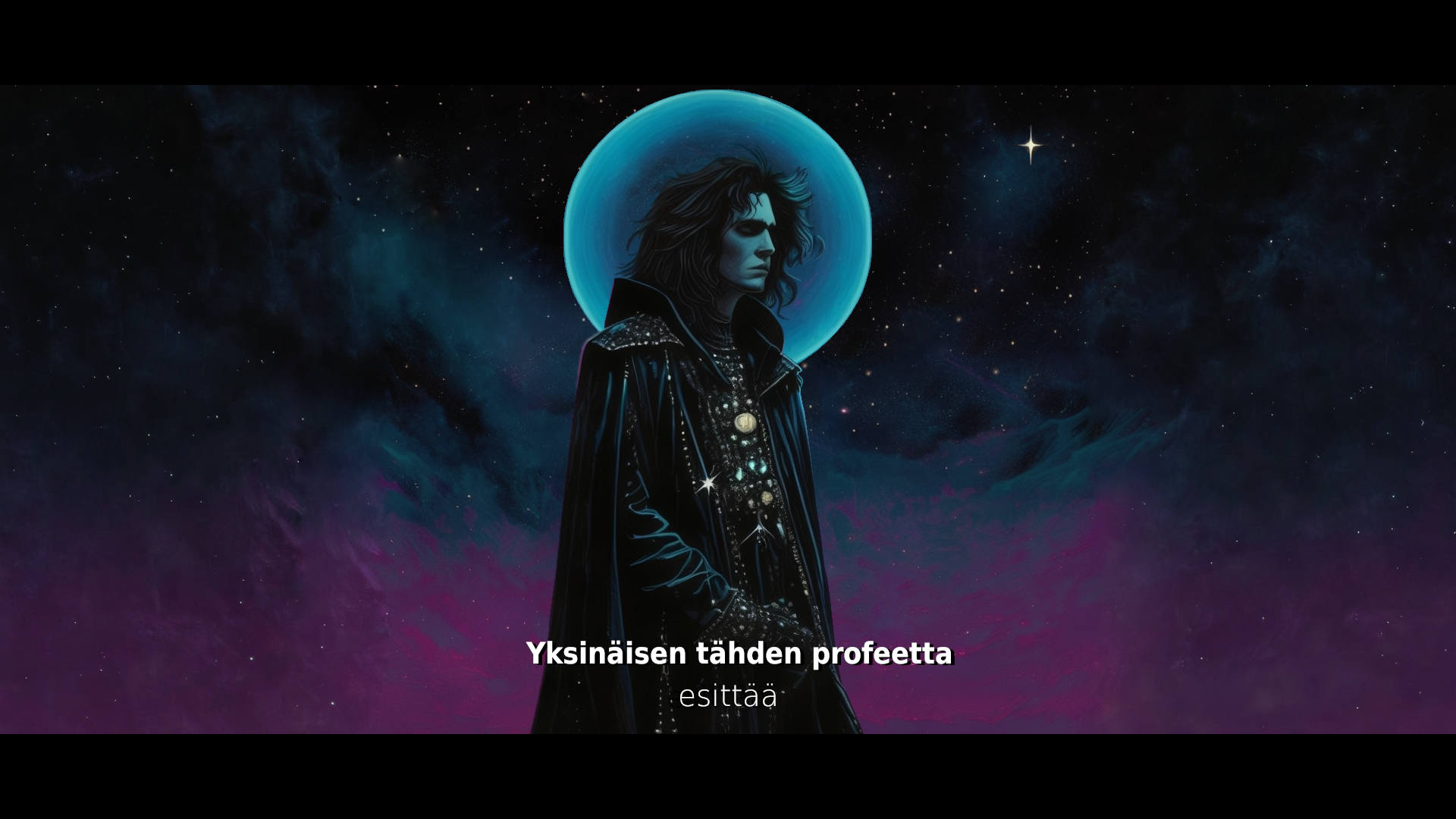
Getting Godot’s default static splash screen localized is a nightmare. One might assume it’s as easy as 1, 2, ,3, just set a remap for it and you’re done, but oh no, of course it isn’t supported.
Furthermore, because all the user space code loads only after the splash screen has already been shown, it complicates things.
And to make the matters even worse, of course any runtime changes to ProjectSettings
are not persistent, so you can’t just set a new path for the boot splash when the locale changes.
However, there is a way.
Project Settings
In the Godot Editor under Project Settings > General > Application > Boot Splash
- Set
Show Image
totrue
. - Set
Image
to your default image.
Under Project Settings > General > Application > Config
set Project Settings Override
to override.cfg
.
Writing to override.cfg
When your locale changes, call:
// Set the splash image to graphics localized to Finnish.
ProjectSettings.SetSetting("application/boot_splash/image", "user://path/to/my/localized/splash_fi.png");
// Save the changed setting to override.cfg.
ProjectSettings.SaveCustom("override.cfg");
This special override.cfg
file will be created in the folder where your game’s executable resides. It will be loaded via the project settings as defined above.
Setting the splashes in user://
Trying to use the splashes from res://
resulted in an utter failure for me. The solution was copying the images to user://
. Of course you can’t just copy them, that would be too simple. But we can write the image files.
You can do this how ever you like, here’s what I did:
private static void TryToCopySplashImagesToUserFolder() {
// Copy the splash screen images to the user folder if they don't exist there.
string splashScreenFolderPath = Paths.GetSplashScreenFolderPath();
string splashScreenFolderFullPathFi = Path.Combine(splashScreenFolderPath, "ProphetOfTheLonelyStar_fi.png");
string splashScreenFolderFullPathEn = Path.Combine(splashScreenFolderPath, "ProphetOfTheLonelyStar_en.png");
// Check if the folder exists.
if (!Directory.Exists(splashScreenFolderPath)) {
Directory.CreateDirectory(splashScreenFolderPath);
}
// Write splash screens to user folder.
// English splash screen. if (!File.Exists(splashScreenFolderFullPathEn)) {
string fileNameEn = "ProphetOfTheLonelyStar_en.png";
string imageFilePathEn = $"res://Textures/SplashScreen/{fileNameEn}";
string writeFilePathEn = Path.Combine(Paths.GetSplashScreenFolderPath(), fileNameEn);
Texture2D imageEn = GD.Load<Texture2D>(imageFilePathEn);
if (!File.Exists(writeFilePathEn)) {
// Write the imageEn to the destination file.
try {
imageEn.GetImage().SavePng(writeFilePathEn);
} catch (Exception e) {
Console.WriteLine($"Splash screen image file could not be written: {writeFilePathEn}");
Console.WriteLine(e);
}
}
}
// Finnish splash screen.
if (!File.Exists(splashScreenFolderFullPathFi)) {
string fileNameFi = "ProphetOfTheLonelyStar_fi.png";
string imageFilePathFi = $"res://Textures/SplashScreen/{fileNameFi}";
string writeFilePathFi = Path.Combine(Paths.GetSplashScreenFolderPath(), fileNameFi);
Texture2D imageFi = GD.Load<Texture2D>(imageFilePathFi);
if (!File.Exists(writeFilePathFi)) {
// Write the imageFi to the destination file.
try {
imageFi.GetImage().SavePng(writeFilePathFi);
} catch (Exception e) {
Console.WriteLine($"Splash screen image file could not be written: {writeFilePathFi}");
Console.WriteLine(e);
}
}
}
}
These were the main points. Fill in the blanks for your own project and you should be good to go.