Seeded Random Numbers
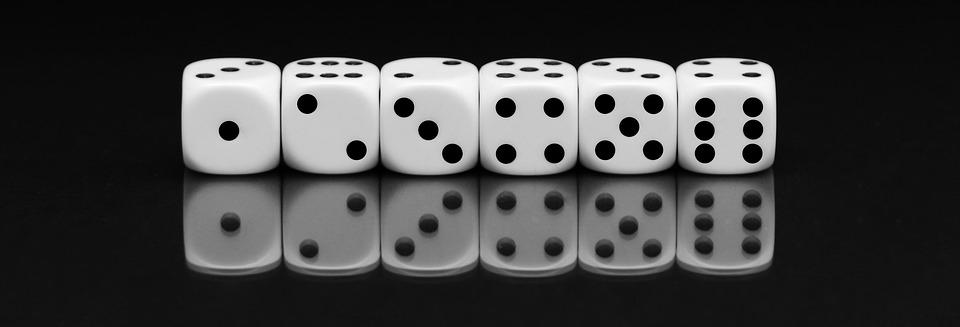
Sometimes you need pseudo-random numbers which are not dependent on time and which are always reliably identical and given in the same order when ever you re-start the application or re-create the Random
generator object.
This can be achieved by giving the Random
generator instance a seed value.
Random random = new Random(1273456);
This is very useful in situations where you, for example, need to generate identical terrain each time your game runs.
The seed value could be shared between client and server or between friends to generate the same world on different machines provided that the generation code remains identical between versions of the application.
Several Random Number Generators Sharing a Seed
Csharp’s random number generator is not thread safe. This means if you need random number generation and you have several threads which need those numbers they can’t share the same instance, it’s not safe to do so.
In such cases you might need to have several random number generators which are based on the same seed to keep the behavior consistent.
A common way to do this is to use a master random number generator and have that generate the seed values for any subsequent random number generators. But this approach has problems even though it’s common. If you do this you will get bi-modal peaks for your range where it’s more likely to get numbers from those peaks - making the random generation less random.
A simple working solution to this problem is to increment your seed by +1 for each new subsequent random number generator like shown in the example below.
using System;
internal class SeededRandomExample {
public Random TerrainRandom;
public Random BiomeRandom;
public Random VegetationRandom;
public int Seed { get; set; } = 481203849;
public SeededRandomExample() {
int terrainSeed = Seed;
TerrainRandom = new Random(terrainSeed);
int biomeSeed = Seed + 1;
BiomeRandom = new Random(biomeSeed);
int vegetationSeed = Seed + 2;
VegetationRandom = new Random(vegetationSeed);
}
}
This solution will give you better and more consistently (pseudo-) random numbers while keeping the random number generators tied to the same seed value.